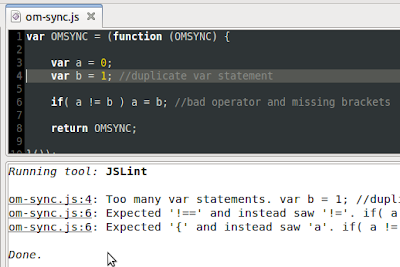
For coding Javascript, I use gedit and several plugins/external tools. One of them is JSLint. Here is a short tutorial get a good integration of JSLint into gedit.
- Install the Rhino Javascript command line engine:
sudo apt-get install rhino - Download and install the rhino version of JSLint. Unfortunately, it is no longer available from the original developer. You can get a copy from the juicer project: https://github.com/cjohansen/juicer/raw/master/lib/jslint/jslint-rhino.js (save link as). Copy the file to: $HOME/.gnome2/gedit/plugins/jslint-rhino.js
- Open gedit to configure JSLint as external tool.
Enable the External Tools plugin and restart gedit.Go to Tools > Manage External Tools and add a new entry. - Copy&Paste the following script into the text area. Make sure that file name and path of the downloaded JSLint file is the same as in this script.
#!/bin/sh
echo "/*jslint onevar: true, undef: true, newcap: true, nomen: true, regexp: true, plusplus: true, bitwise: true, browser: true, maxerr: 50, indent: 4, white: false */" > /tmp/jslint-me.js
cat $1 >> /tmp/jslint-me.js
result=$(js $HOME/.gnome2/gedit/plugins/jslint-rhino.js /tmp/jslint-me.js)
rm /tmp/jslint-me.js
js $HOME/.gnome2/gedit/plugins/jslint-rhino-text-replace.js "$GEDIT_CURRENT_DOCUMENT_NAME" $result > /dev/stdout - Select a shortcut (Ctrl+J
et the other options to) and s
Save: Nothing
Input: Current Selection (default to document) - Finally you need to copy another command line script to reformat the JSLint output. It will allow you to click the error messages in gedit, jumping to the corresponding line in your code. Copy the following script to: $HOME/.gnome2/gedit/plugins/jslint-rhino-text-replace.js. Make sure that file name and path of the text-replace script file is the same as in the gedit External Tools script.
var newArgs = [], errorPrefix, i = 1, lastErrorIndex = -1, fileName = "";
var len = arguments.length, fileName = arguments[0];
while (i < len) {
if (arguments[i] === "Lint" && arguments[i+1] === "at"){ //new Lint starting
errorPrefix = fileName + ":" + (arguments[i+3]-1) + ":";
newArgs.push(errorPrefix);
lastErrorIndex = newArgs.length-1;
i += 6;
}
newArgs[lastErrorIndex] += " " + arguments[i];
i += 1;
}
for(i = 0; i < newArgs.length; i += 1) {
print(newArgs[i]);
}
if(newArgs.length === 0) {
print(fileName + ": Excellent! Your JS code is clean and shiny.");
}
//
// Copyright (C) 2011 by Uwe Jugel, uwe.jugel@gmail.com
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
//
// Info:
// gedit support referencing and navigating from shell output lines to source
// code lines if the output follows the "filename:linenumber:message" pattern
// This script removes some verbosity from the JSLint error messages and
// reformats them to support this pattern.
//
// Use this script after calling JSLint from gedit External Tools.
//
// Usage:
// js PATH_TO/jslint-rhino-text-replace.js FILENAME JSLINTOUTPUT...
//
Juve
Comments